Last year, when it was still possible, I attended a ReactDay Meetup in Berlin and was astounded by an awesome talk by an amazing speaker & a mentor, Tejas Kumar. His talk was called "Destructuring React" and instead of a bunch of slides, he amazed everybody by live-coding "Poor Man's React" (his words).
Starting from scratch, he reverse-engineered some React functions like createElement
& useState
building them in vanilla JS. This idea struck me making me believe that it is possible to do the same for many other libraries. I am finally putting my idea into words & code.
I am going to reverse engineer the styled-components library with some basic features. Here's how it goes:
index.html
To begin with, I am going to create a "styled" component and render it using React. Remember, I did not install the styled-components
library, because I am going to create it!
index.tsx
Guess what happens when I serve this? I get an ERROR!

To create my own styled-components library, I will create a file styled.tsx
, but to even start writing code there, we need to understand how the styled-components
API works.
Deciphering the way we write a styled component, styled
is a function which accepts "tag name" as an argument & returns another function that accepts a "tagged template". According to MDN:
"Tags allow you to parse template literals with a function. The first argument of a tag function contains an array of string values. The remaining arguments are related to the expressions."
To test this logic, I am going to create a function called styled that just logs all the arguments that are passed to it.
styled.tsx
Testing this by including it in index.tsx
, I get the following in console:
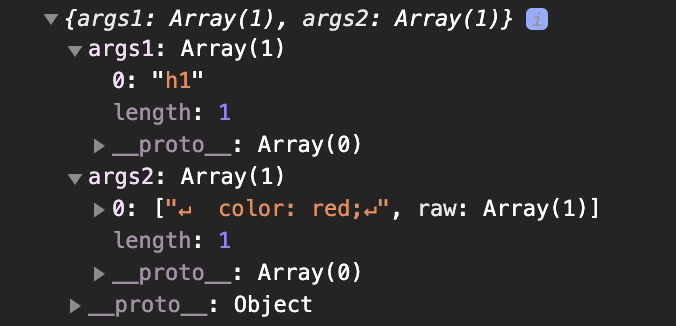
Since I am trying to render this component, I also get an error from React.
Recognizing the console.log, the first argument is the HTML tag (Tag
) and the second argument is the array containing style strings (styleArr
).
I am now going to return a functional component that renders the original HTML tag & persists all props. I am also going to de-structure the className
prop and rename it to originalClassName
.
styled.tsx
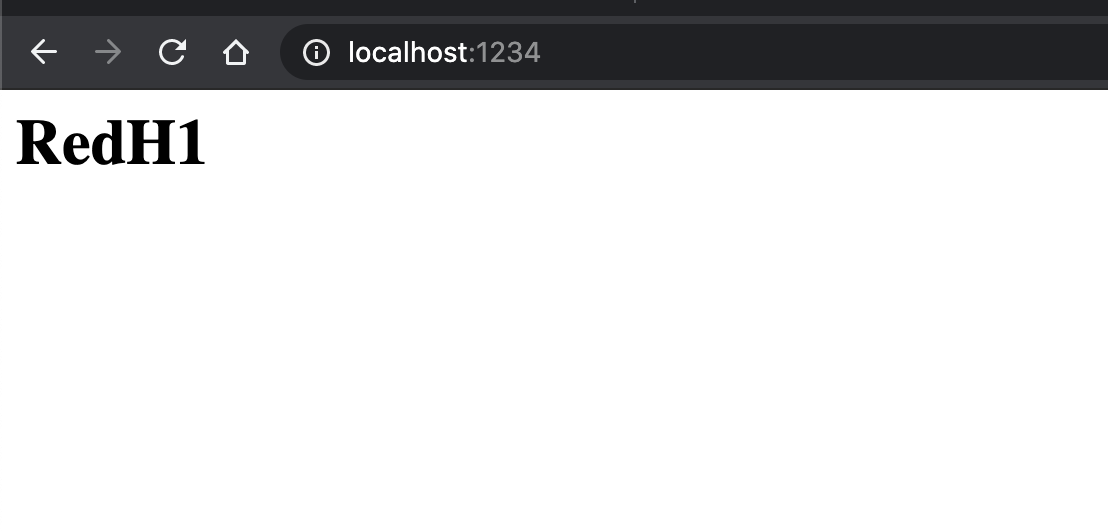
I must now take care of the styles and apply them to the h1
element. If you have ever noticed the generated HTML/CSS when using the original styled-components, it generates classNames
and outputs all css styles in an internal stylesheet. Trying to recreate that, I am gonna focus of the following features:
There should only be one style tag in head section of the document.
All styled-components having same css should reuse the className.
The className should be generated using the css without considering the tag name.
The components should be chain-able (a component can use another component as a base).
styled.tsx
I picked up a hashing function from StackOverflow that returns a numerical string, therefore, I prefix it with "style".
Rest is rather straightforward, and the comments should be self-explanatory.
Check out the whole code with some more examples here: https://github.com/shreyansqt/reverse-engineering-styled-components.
You must be wondering, what about the styled.h1
syntax of styled-components. Since all functions are objects in javascript, we can simply create object properties for all tag names as follows:
I am off to some more adventures of similar sort. If you have any comments or would like to discuss this post, reach out to me on https://twitter.com/shreyansqt & let's grab a virtual coffee.